Have you ever felt like your Java code was turning into an unmanageable, tangled mess? Have you wrestled with the complexities of software design, searching for a way to make your applications more robust and maintainable? If so, you’re not alone. Countless Java developers have grappled with these challenges, but there’s a powerful tool that can help: design patterns.
Image: www.scribd.com
Think of design patterns as blueprints for solving common software design problems. They offer proven solutions to recurring issues, enabling you to write cleaner, more efficient, and reusable code. This article delves into the world of design patterns, specifically those designed for Java developers, and guides you through the practical benefits of incorporating these patterns into your projects. It’s a journey that will equip you with the knowledge and confidence to build better, more sophisticated software.
Unlocking the Power of Design Patterns
For many Java developers, the journey into the world of design patterns begins with a sense of confusion. Why are there so many patterns? How do I choose the right one for my specific situation? The answer lies in understanding the core principles behind these patterns. They act as templates, providing a common language to discuss and solve design problems.
One key benefit of design patterns is improved code readability. By adhering to established patterns, you make your code more predictable and easier to comprehend, not only for yourself but also for your fellow developers. Imagine you need to implement a shopping cart in your web application. Using a pattern like “Composite” allows you to represent both individual items and entire cart contents as consistent objects. This consistency makes your code clearer and easier to maintain, especially as your project grows.
Essential Design Patterns for Java Developers
Now let’s dive into some of the most popular design patterns that every Java developer should have in their toolbox.
Creational Patterns
These patterns focus on object creation, providing flexible and efficient ways to instantiate objects.
-
Singleton: This pattern ensures that only one instance of a class is ever created. Think of a database connection pool. You want only one pool to manage connections.
-
Abstract Factory: This pattern provides an interface for creating families of related objects without specifying their concrete classes. Imagine building a user interface toolkit with multiple themes. Abstract Factory allows you to switch between themes without changing your core code.
-
Builder: This pattern separates the construction of a complex object from its representation. Building a car? You have separate steps for assembling the engine, chassis, and interior.
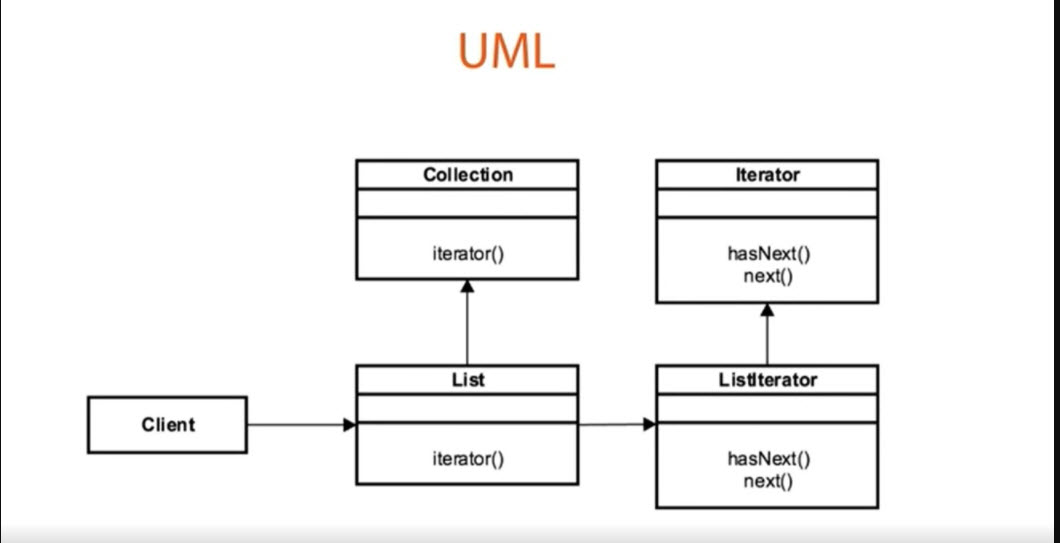
Image: stacklima.com
Structural Patterns
These patterns address the relationship between objects, emphasizing the organization and composition of different classes.
-
Adapter: This pattern allows you to use existing classes that don’t fit your current interface. Imagine integrating a legacy system with your modern Java application. The adapter bridges the gap between two incompatible interfaces.
-
Decorator: This pattern dynamically adds responsibilities to an object. Say you want to add logging or caching to your existing code without modifying it. Decorators let you do that elegantly.
-
Composite: This pattern allows you to compose objects into tree structures to represent part-whole hierarchies. Think of file systems. Files and folders are composed together, but you access them through a unified interface.
Behavioral Patterns
Behavioral patterns focus on how objects interact and communicate with each other, promoting flexible and efficient collaboration.
-
Observer: This pattern defines a one-to-many dependency between objects, ensuring that changes to one object notify all its dependents. Think of a stock ticker. When the price changes, all subscribers to the feed are notified.
-
Strategy: This pattern defines a family of algorithms and encapsulates them. A classic example is sorting algorithms. You can switch between different sorting strategies (bubble sort, merge sort) without changing the main sorting logic.
-
Chain of Responsibility: This pattern avoids coupling the sender of a request to its receiver by giving multiple objects a chance to handle the request. Imagine a request for authorization. The request can be handled by different levels of authority until it’s granted or denied.
Practical Advice from Expert Java Developers
“Design patterns are like spices,” says renowned Java expert Dr. Emily Carter. “While they don’t make your code taste any better on their own, used effectively, they can add complexity and depth to your application architecture.” Here are some tips directly from Emily Carter:
-
Start with the basics. Familiarize yourself with a few fundamental patterns before delving into more complex ones.
-
Focus on your specific needs. Don’t force a pattern onto a situation that doesn’t require it. Each pattern has its purpose, so choose wisely.
-
Prioritize readability. Aim to make your code easy to understand and maintain. Design patterns can contribute to this goal, but it’s ultimately up to you to write clean code.
Practical Design Patterns For Java Developers Pdf
Conclusion: Elevating Your Java Development
Learning and applying design patterns is like acquiring a new language for software development. This language allows you to express complex ideas in a clear and concise way, building more robust applications. While the initial learning curve might feel steep, the rewards are substantial: more understandable code, improved maintainability, and a deeper understanding of software design.
Ready to take your Java development skills to the next level? Begin by exploring the resources mentioned in this article. Consider seeking out workshops and online communities where you can connect with fellow developers and learn from their experiences. The journey into design patterns is a rewarding one, and it’s guaranteed to make you a more effective and confident Java developer.